Sidekiq: An introduction to background job processing
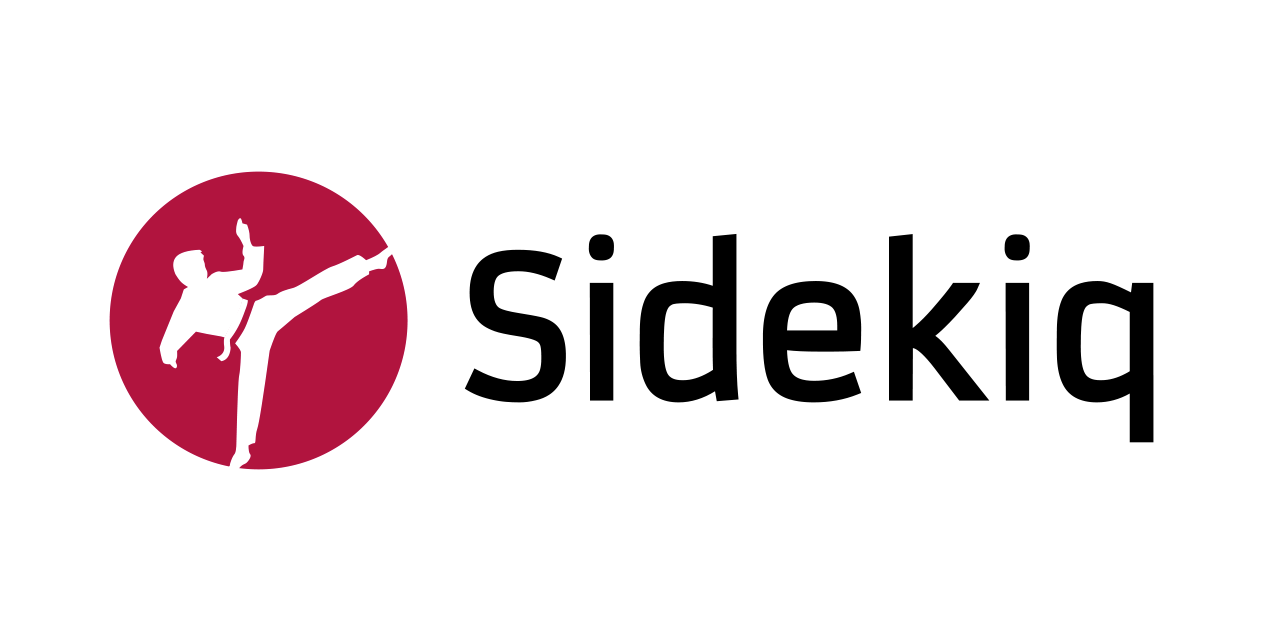
Processing millions and thousands of records is a time-consuming task that cannot be completed quickly. The user must wait for the process to complete, which can be a lengthy and inconvenient process if done in the foreground.
Sidekiq is a background job processing system for Ruby applications. It allows developers to asynchronously execute long-running or resource-intensive tasks in the background, freeing up the application to handle other requests in the meantime.
First, let’s explore how to integrate Sidekiq into our existing application:
To use Sidekiq, we first need to install its gem. We can do this by adding the following line to our Gemfile
:
1
gem 'sidekiq'
After this, run bundle install
Add Sidekiq Worker:
With Sidekiq added to our project, the next step is to create a Sidekiq worker that can perform background jobs.
To create a Sidekiq worker, we should add it to the app/workers
directory. As an example, let’s create a ButTimeWorker
that takes some data as input, sleeps for a specific time, and then prints some output as follows:
1
2
3
4
5
6
7
8
9
10
11
# app/workers/buy_time_worker.rb
class BuyTimeWorker
include Sidekiq::Worker
def perform(name, sec)
p "Hey #{name} you are going to sleep for #{sec} seconds"
sleep(sec)
p "After a long nap"
end
end
This sidekiq worker takes name and sec as arguments, prints the name
after that sleeps for sec
seconds, and prints After a long nap
.
Calling sidekiq worker:
Let’s consider we have a team model as follows:
1
2
3
4
5
6
7
8
9
10
11
12
13
14
# app/models/team.rb
# == Schema Information
#
# Table name: teams
#
# id :bigint not null, primary key
# name :string
# rating :integer
# created_at :datetime not null
# updated_at :datetime not null
#
class Team < ApplicationRecord
end
Controller:
1
2
3
4
5
6
7
8
9
10
11
12
13
14
15
16
17
18
19
20
# app/controllers/api/v1/teams_controller.rb
class Api::V1::TeamsController < ApplicationController
def index
teams = Team.all.select(:id, :name, :rating)
render json: { data: teams }
end
def show
team = Team.where(id: team_params[:id])
.select(:id, :name, :rating)
BuyTimeWorker.perform_async(team.first.name, team.first.rating)
render json: { data: team }
end
private
def team_params
params.permit(:id)
end
end
It’s important to note that we’re calling our worker in the
show
action, and this call is made asynchronously. As a result, we can see the rendered team’s data as JSON while the worker performs its operations in the background.
Routes:
1
2
3
4
5
6
7
8
9
# config/routes.rb
Rails.application.routes.draw do
namespace :api do
namespace :v1 do
resources :teams, only: [:index, :show]
end
end
end
To call our worker, we need to make a request to the following URL: /api/v1/teams/:id
. As soon as this URL is hit, the show
action is called, triggering the Sidekiq worker to begin processing in the background.
In order to see all these things in action, we need to start following things:
Rails server
Redis server
Sidekiq
Output:
1
2
3
4
2023-03-05T12:13:23.217Z pid=11935 tid=11t5jub class=BuyTimeWorker jid=94b8bc2d6afccd72ec101f64 INFO: start
"Hello world"
"Hey Ind you are going to sleep for 9 seconds"
"After long nap"
You’ll see output like this 👆🏼.
In summary, Sidekiq is an excellent choice for implementing background processing in Ruby on Rails applications. With its simple integration and powerful capabilities, Sidekiq can help improve the performance and responsiveness of your application. Give it a try and see how it can benefit your project! 🥂🍻